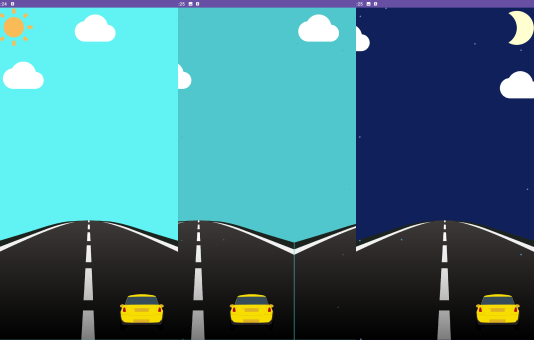
How to use ViewPager2 with animations in Android?
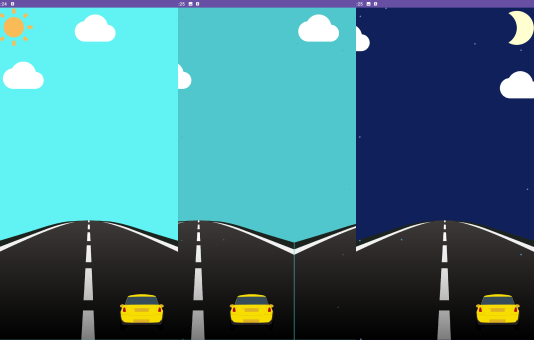
ViewPager2 is a component in the AndroidX Library, used to display items (fragments, views) in an interface that can slide horizontally or vertically in an Android application. So when we use Viewpager we can add different layouts in one activity and this can be done using fragments. Famous apps like WhatsApp, Snapchat use Viewpager. You can find more details about ViewPager2 here.
Following are the steps to create ViewPager2 with animation in Android.
Step 1: Create a New Project
create a new project in Android Studio with kotlin language
Step 2: Creating Fragments and Designing the UI
To create a new Fragment in your project, proceed as follows:
1. Click File-> New-> Fragment-> Fragment(Blank)-> Finish
note: you need to create at least 2 fragments
2. Designing the UI for Fragments
3. Designing the UI for MainActivity
create views to perform animations
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <androidx.viewpager2.widget.ViewPager2 android:id="@+id/pager" android:layout_width="match_parent" android:layout_height="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <View android:id="@+id/night_dots" android:layout_width="match_parent" android:layout_height="0dp" android:alpha="0" android:background="@drawable/bg_dots_night" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageView android:id="@+id/img_cloud_night" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="30dp" android:layout_marginTop="30dp" android:src="@drawable/ic_cloud" android:translationX="-400dp" app:layout_constraintEnd_toStartOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageView android:id="@+id/img_moon" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_moon" android:translationY="-600dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageView android:id="@+id/img_cloud_night2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:src="@drawable/ic_cloud" android:translationX="400dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toBottomOf="@id/img_moon" /> <ImageView android:id="@+id/img_sunny" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_sunny" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageView android:id="@+id/img_cloud1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="30dp" android:layout_marginTop="20dp" android:src="@drawable/ic_cloud" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@id/img_sunny" /> <ImageView android:id="@+id/img_cloud" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginEnd="90dp" android:src="@drawable/ic_cloud" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toEndOf="@+id/img_sunny" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
note: translationX and translationY is set the first position
Step 3: Initialize ViewPager2, manage page scroll action and change ViewPager2 background color on scroll
1. First you need to call the constants in the companion object:
companion object { private const val NUM_PAGES = 2 private const val MEDIUM_DISTANCE = 400 private const val FAR_DISTANCE = 600 }
2. Next you need to create a new class to define extends from FragmentStateAdapter():
Your adapter classes also need to override 2 different methods:
– getItemCount(): return the quantity pager.
– createFragment(position: Int): return position to display at fragment.
3. create a function initialize ViewPager2, configure adapter and manage ViewPager2’s scrolling actions:
4.create a function to change the background color of ViewPager2 when scrolling the page:
Declare colors and evaluator
private var colors: IntArray= intArrayOf( this.getColor(R.color.blue), this.getColor(R.color.night), ) // This evaluator can be used to perform type interpolation between integer values that represent ARGB colors private var evaluator: ArgbEvaluator = ArgbEvaluator()
Change Page Background Color
private fun changePageBackgroundColorOnSwipe( position: Int, pagerAdapter: ScreenSlidePagerAdapter, positionOffset: Float ) { if (position < pagerAdapter.itemCount - 1 && position < colors.size - 1) { binding.pager.setBackgroundColor( (evaluator.evaluate( positionOffset, colors[position], colors[position + 1] ) as Int) ) } else binding.pager.setBackgroundColor(colors[colors.size - 1]) }
After writing, put changePageBackgroundColorOnSwipe() function inside onPageScrolled().
Step 4: ViewPager2 with animations
Show and hide animations using the view.animate().alpha(positionOffset).setDuration(duration).start() method
complete code snippet for onPageScrolled()
override fun onPageScrolled(position: Int,positionOffset: Float,positionOffsetPixels: Int) { super.onPageScrolled(position, positionOffset, positionOffsetPixels) changePageBackgroundColorOnSwipe(position, pagerAdapter, positionOffset) showDotsAndStars(position, positionOffset) }
Step 5: Run to view result