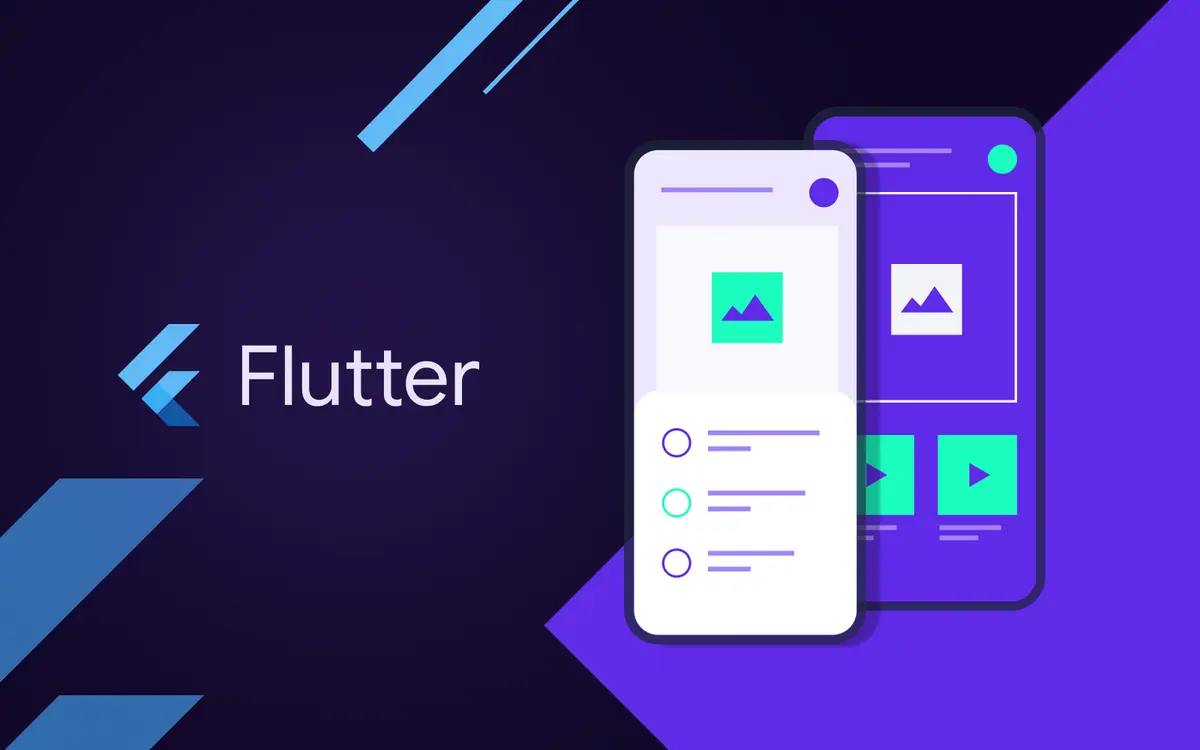
App development with flutter
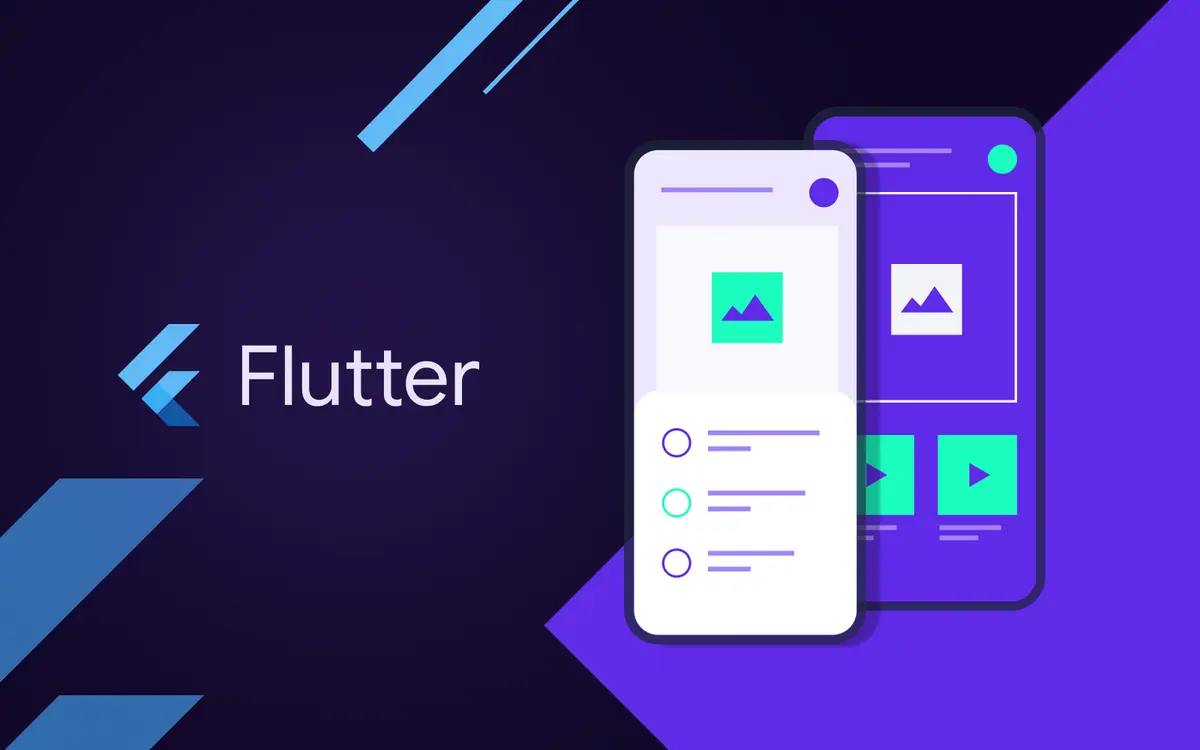
Introduction
Flutter is an open source framework by Google for buidling beautiful, natively complied, multi-platform applications from a single codebase.
Overview Flutter App
Overview for project
Flutter App is a multi-platform development project combining content management and people management
Flutter App content management : Flutter App manages of the relief product warehouse, the shelter. In addition, there are documents related to above items.
Flutter App people management : Flutter App manages the people in evacuation place, manages the lower personel.
Supported Platforms : Android(phone, tablet), iOS(iPhone, iPad), windows, webapp Why using Flutter for develop Flutter App
With a project developed on multiple platforms. To optimize development time, we should choose a cross- platform framework with less code, low development time. But still ensure the performance and reliability of the product.
One of the popular framework that can be used on many platform(including windwows) is Flutter
Pros of Flutter app development
Pros of Flutter app development
1. Development Time
- The first Reason for choosing Flutter is development time. Flutter is based on dart language and if you are familiar with java and similar programming language it is really easy to learn Flutter. In Flutter logic and UI will be written in the same code-base. unlikely in native android had to learn XML-based UI and it is really hard to familiar with new layout formats. But in a Flutter, all UI elements are available as widgets.
- Due to this reason, it is really efficient and within a minimum time period can do MVP product to the market.
2. Same Code, All Platforms
- At the early stage of mobile development around a few years back we had the biggest issue was managing two mobile developers for android and ios. It is really hard to manage two code-base for android and IOS because to do the change in each project takes time and it is an error-prone task.
- Flutter’s code reusability allows you to write just one codebase and use it on not only for mobile Android and iOS but even for web, desktop and more. This cuts development time significantly, removes cost and enables you launch your app that much faster.
3. App Performance
- When rating one of language or framework. Maybe the best way is compare language or framework with other language or framework in same function. One of the other popular cross-platform framework is React-native is how to render the UI for each platform.
- With android, when it render view to device. The Android system libraries provide components responsible for drawing themselves to a Canvas object, which Android can then render using Skia a graphics engine written in C/C++ that call the CPU/GPU to complete the drawing on the device.
- Cross-platform frameworks typically work by creating an abstraction layer on top of the underlying Android/iOS native UI libraries, and trying to solve problems specfic to each platform.
- As you can see on the picture. React Native communicates with the platform throught a bridge, Flutter communicates with platform directly. That’s what makes the difference in performance between these two frameworks.
- On the sidelines:
4. Rich UI features
- In Native android development biggest challenge was to be familiar with XML layout to build the UI components. Flutter has matured UI library and it can be easily added to the project. The main advantage of the Flutter is It support Hot Reload the UI components.
- All the UI libraries come up as a widget, due to this reason within minimal code we can include the library and test.
- For example, assume needs to implement a full circle shape image. In a native way had to write so many XML files to do that. But in Flutter, just a few lines of code are enough.
- Ex: Circle Image:
ClipRRect(
borderRadius: BorderRadius.circular(8.0), child: Image.network(
subject['images']['large'],
height: 150.0,
width: 100.0,
), )
- These are the few sample apps UI that is written in Flutter
Cons of Flutter app development
1. Large file size because of the widgets
2. Complex updating
Updating programming requirements in operating systems requires updating Flutter modules. Since the modules are integrated as fixed elements in the program, the latter must also be recompiled and reinstalled on the devices.
3. Limited set of tools and libraries
Flutter is a quite new development framework, so in some cases you may not be able to get the desired functions in the current library. Flutter will take some time to create certain tools, expand functionality and develop the community.
Libraries used in the Flutter App
1. getx
- GetX is an extra-light and powerful solution for Flutter. It combines high-performance state management, intelligent dependency injection, and route management quickly and practically.
- In Flutter App, I’m using GetX for state management, dependency injection and route management State management : It controls the screen’s state, the data call process, the screen’s lifecycle
abstract class ViewModelBase extends GetxController { final logger = Logger();
final status = Status.normal.obs;
void listenConnectivityStatus() {
}void cancelConnectivitySubscription() {
// subscription.cancel(); }
void onInitView() {} void onDisposeView() {} void onConnected() {} void onDisconnect() {}
}
- Dependency Injection : Init service, controller,…
class AdminHomeBinding extends Bindings { @override
void dependencies() {
Get.lazyPut<AdminHomeViewModel>( () => AdminHomeViewModel(
userService: Get.find<UserServiceImpl>(), freeItemService: Get.find<FreeItemServiceImpl>(), informationService: Get.find<InformationServiceImpl>(), shelterService: Get.find<ShelterServiceImpl>(), shelterDataService: Get.find<ShelterDataServiceImpl>(), staffService: Get.find<StaffServiceImpl>(), messageService: Get.find<ChatServiceImpl>(),
), );
} }
- Route : management router in app
GetPage(
name: Routes.admin_home, page: () => AdminHomeView(), binding: AdminHomeBinding(),
), GetPage(
name: Routes.client_home, page: () => ClientHomeView(), binding: ClientHomeBinding(),
),
2. dio
A powerful Http client for Dart, which supports Interceptors, Global configuration, FormData, Request Cancellation, File downloading, Timeout etc.
- Call API
var dio = Dio(); // with default Options
// Set default configsdio.options.baseUrl = ‘https://www.xx.com/api’; dio.options.connectTimeout = 5000; //5s dio.options.receiveTimeout = 3000;
// or new Dio with a BaseOptions instance. var options = BaseOptions(
baseUrl: ‘https://www.xx.com/api’, connectTimeout: 5000, receiveTimeout: 3000,
);
Dio dio = Dio(options);
- Download file
response = await dio.download('https://www.google.com/', './xx.html');
3. connectivity_plus
This plugin allows Flutter apps to discover network connectivity and configure themselves accordingly. It can distinguish between cellular vs WiFi connection.
case ConnectivityResult.wifi: onConnected(); logger.d(‘ConnectivityResult.wifi’); break;Future<void> listenConnection(ConnectivityResult result) async { switch (result) {
case ConnectivityResult.mobile: logger.d(‘ConnectivityResult.mobile’); onConnected();
break;
case ConnectivityResult.none: logger.d(‘ConnectivityResult.none’); onDisconnect();
break;
case ConnectivityResult.bluetooth: // TODO: Handle this case. break;
case ConnectivityResult.ethernet: // TODO: Handle this case. break;
case ConnectivityResult.vpn: // TODO: Handle this case. break;
} }
4. geolocator
A Flutter geolocation plugin which provides easy access to platform Features:
- Get the last known location
- Get the current location of the device
- Get continuous location updates
- Check if location services are enabled on the device
- Calculate the distance (in meters) between two geocoordinates Calculate the bearing between two geocoordinates
Example:
/// Determine the current position of the device. ///import 'package:geolocator/geolocator.dart';
/// When the location services are not enabled or permissions
/// are denied the `Future` will return an error. Future<Position> _determinePosition() async {
bool serviceEnabled; LocationPermission permission;
// Test if location services are enabled.
serviceEnabled = await Geolocator.isLocationServiceEnabled(); if (!serviceEnabled) {
// Location services are not enabled don’t continue
// accessing the position and request users of the
// App to enable the location services.
return Future.error(‘Location services are disabled.’);
}
permission = await Geolocator.checkPermission(); if (permission == LocationPermission.denied) {
permission = await Geolocator.requestPermission(); if (permission == LocationPermission.denied) {
// Permissions are denied, next time you could try
// requesting permissions again (this is also where
// Android’s shouldShowRequestPermissionRationale
// returned true. According to Android guidelines
// your App should show an explanatory UI now.
return Future.error(‘Location permissions are denied’);
} }
if (permission == LocationPermission.deniedForever) {
// Permissions are denied forever, handle appropriately. return Future.error(
‘Location permissions are permanently denied, we cannot request permissions.’); }
// When we reach here, permissions are granted and we can // continue accessing the position of the device.
return await Geolocator.getCurrentPosition();
}
5. realm
- Realm is a mobile database that runs directly inside phones, tablets or wearables. This repository holds the source code for the Realm SDK for FlutterTM and DartTM.
- Realm is RAM database
- Features:
- Mobile-first: Realm is the first database built from the ground up to run directly inside phones, tablets, and wearables.
- Simple: Realm’s object-oriented data model is simple to learn, doesn’t need an ORM, and the API lets you write less code to get apps up & running in minutes.
- Modern: Realm supports latest Dart and Flutter versions and is build with sound null-safety.
- Fast: Realm is faster than even raw SQLite on common operations while maintaining an extremely rich feature set.
- Example:
import 'dart:io';
import 'package:flutter/material.dart'; import 'package:realm/realm.dart';
part ‘main.g.dart’;
@RealmModel() class _Car {
late String make; String? model;
int? kilometers = 500; _Person? owner;
}
@RealmModel() class _Person {
late String name;
int age = 1; }
void main() { print(“Current PID $pid”); runApp(MyApp());
}
class MyApp extends StatefulWidget { @override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> { late Realm realm;
_MyAppState() {
final config = Configuration.local([Car.schema, Person.schema]); realm = Realm(config);
}
int get carsCount => realm.all<Car>().length;
@override
void initState() {
var myCar = Car(“Tesla”, model: “Model Y”, kilometers: 1);
realm.write(() {
print('Adding a Car to Realm.');
var car = realm.add(Car("Tesla", owner: Person("John"))); print("Updating the car's model and kilometers"); car.model = "Model 3";
car.kilometers = 5000;
print(‘Adding another Car to Realm.’); realm.add(myCar);
print(“Changing the owner of the car.”); myCar.owner = Person(“me”, age: 18);print(“The car has a new owner ${car.owner!.name}”);
});
print(“Getting all cars from the Realm.”);
var cars = realm.all<Car>();
print(“There are ${cars.length} cars in the Realm.”);
var indexedCar = cars[0];
print(‘The first car is ${indexedCar.make} ${indexedCar.model}’);
print(“Getting all Tesla cars from the Realm.”);
var filteredCars = realm.all<Car>().query(“make == ‘Tesla'”); print(‘Found ${filteredCars.length} Tesla cars’);
super.initState(); }
@override
Widget build(BuildContext context) {
return MaterialApp( home: Scaffold(
appBar: AppBar(
title: const Text(‘Plugin example app’),
),
body: Center(
child: Text(‘Running on: ${Platform.operatingSystem}.\n\nThere are $carsCount cars in the Realm.\n’), ),
), );
} }
- Benchmark(Realm & SQLite)
6. google_mlkit
A Flutter plugin to use Google’s ML Kit Text Recognition
to recognize text in any Chinese, Devanagari, Japanese, Korean and Latin character set.
7. stash_hive
- Hive key-value store extension for the stash caching API. Provides support to store vaults and caches in the Hive database
- Example we can cache response api to hive, and using when disconnect network
8. wechat_camera_picker & wechat_assets_picker
- An audio/video/image picker in pure Dart which is the same with WeChat, support multi picking, custom item and delegates override.
resultList = await AssetPicker.pickAssets( context,
pickerConfig: AssetPickerConfig(requestType: RequestType.image,
maxAssets: MAX_ASSETS,
textDelegate: CustomPickerTextDelegate(), specialItemPosition: SpecialItemPosition.prepend, specialItemBuilder: (_, __, ___) {
return GestureDetector(behavior: HitTestBehavior.opaque, onTap: () async {
final AssetEntity? result = await CameraPicker.pickFromCamera( context,
pickerConfig: CameraPickerConfig(
enableAudio: false,
textDelegate: CustomCameraPickerTextDelegate()), );enableRecording: false,
if (result != null) { Navigator.of(context).pop(<AssetEntity>[result]);
} },
child: const Center(
child: Icon(Icons.camera_enhance, size: 42),
), );
}),
- Result:
9. Other(Libraries for UI)