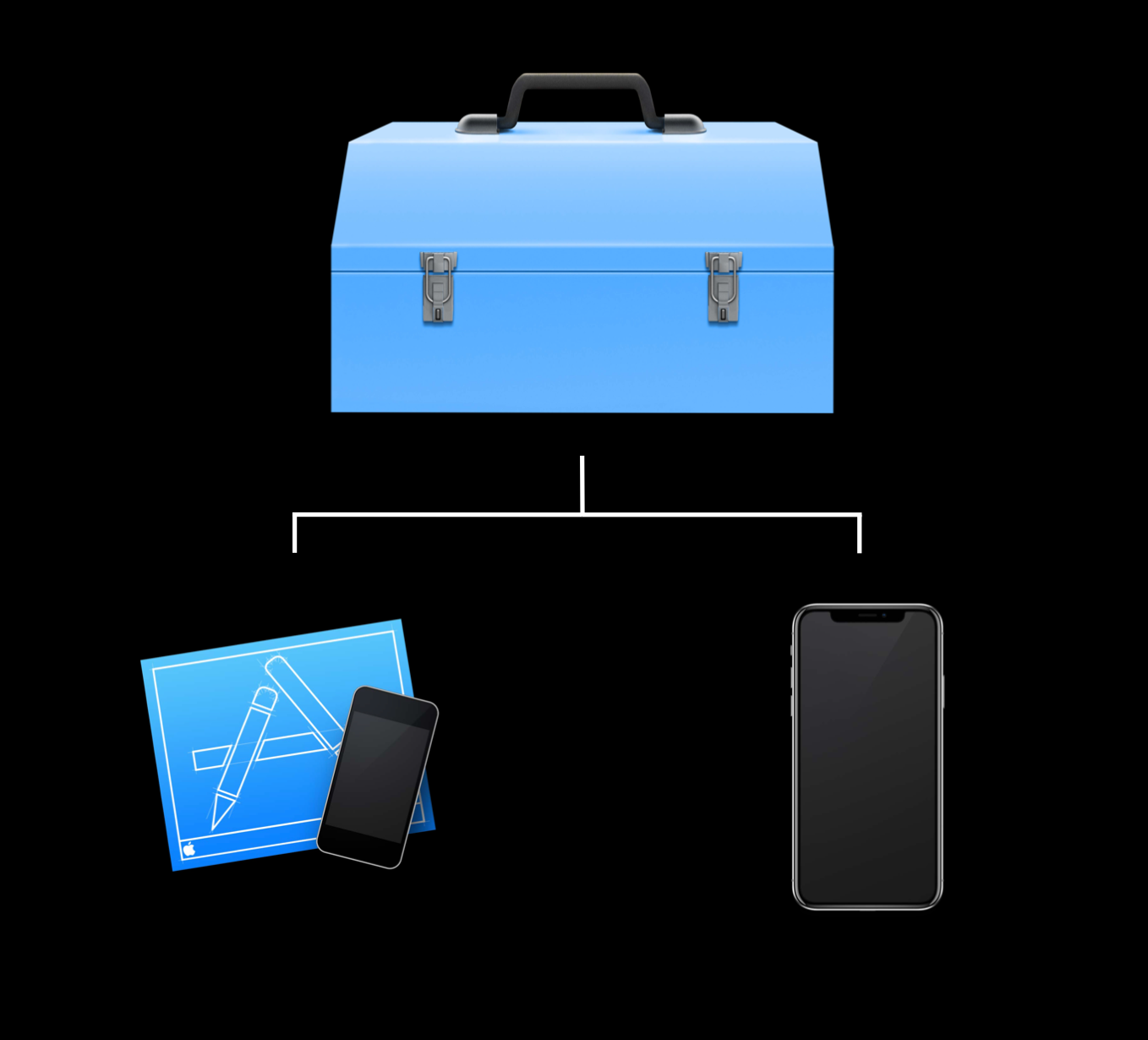
How to build xcframework with Xcode?
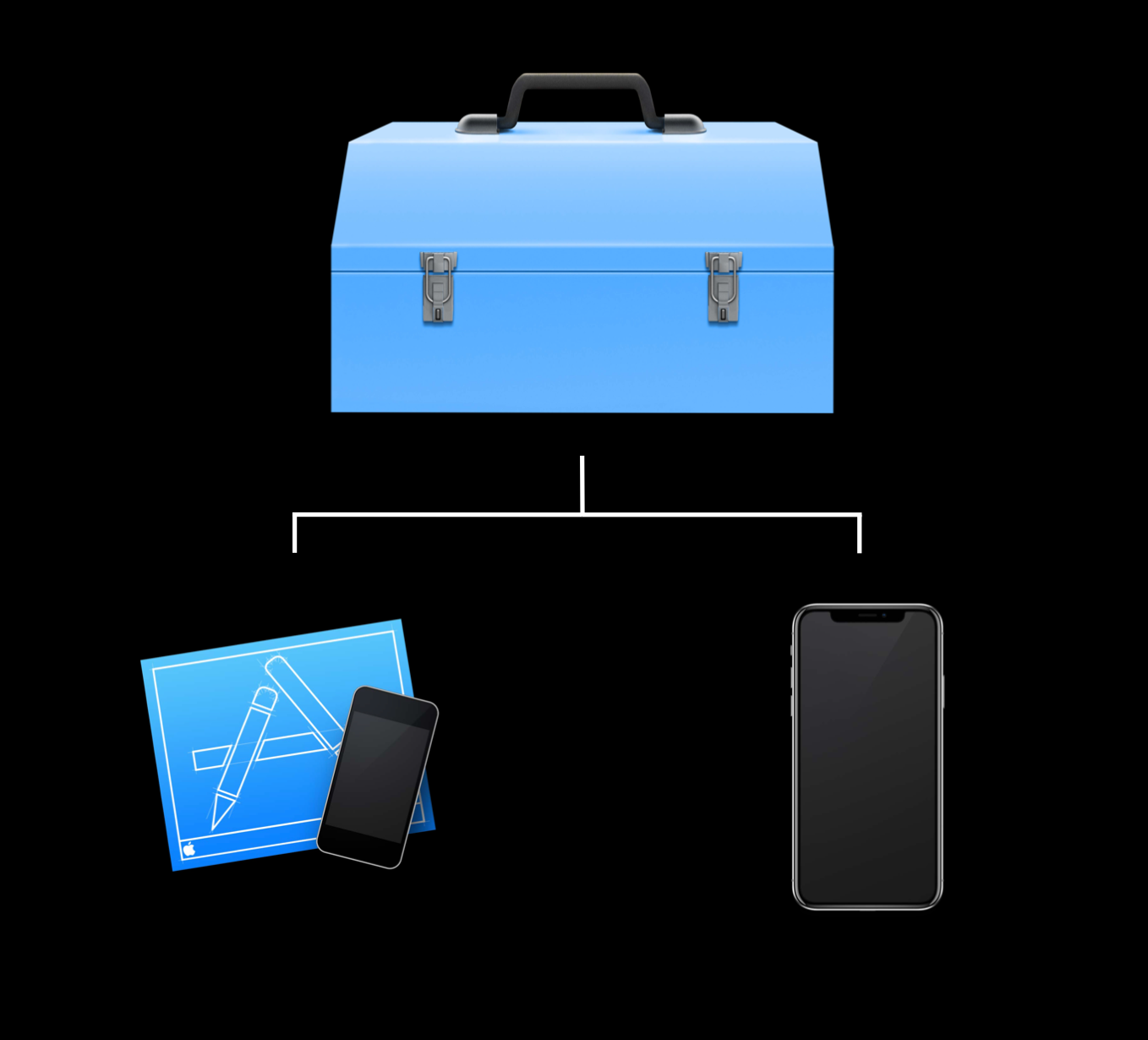
In this article I’ll cover how you can build your framework and test it using xcode.
Create the Framework source code
Open xcode and start with a framework project.
I’ll name mine SimpleMath, you can choose whatever you want, and I’ll save it to my desktop so I can easily access it later when I’m building the xcframework.
Let’s create a new swift file, title it SimpleAlgorithms, the following class will contain some mathematical functions.
As you can see I’ve added public access modifier to be able to access that class from outside the framework, (ie from our project when we import this framework).
Here is thing that you need to know about xcframeworks, they are pre-compiled code (binary code) that you distribute for others.
Xcode builds framworks for specific architectures, so xcode will build framework for simulators and another one for iOS devices and another one for macOS and tvOS.
In most of the cases when you distribute your framework to your client you won’t give them separate frameworks and they replace when they run on simulator or iPhone devices, at least not in 2020
Previously we would create all the frameworks that we need and then use LIPO tool to help us combine all these frameworks in one universal framework .framework and distribute it.
But since apple has introduced the new xcframework, we don’t need LIPO anymore.
So let us see how to create it.
Generating the .xcframwork for simulators and devices
Start by enabling Build Libraries for Distribution from build settings in xcode.
Then, open up a terminal window and navigate to your project directory, in my case it is saved on my desktop
NOTE: All the following commands are for SimpleMath if you named you project something differently you have to adjust all of them with you framework name.
cd ~/Desktop/SimpleMath/
and write the following command, which will create a framework that can run on simulators.
xcodebuild archive \
-scheme SimpleMath \
-archivePath ~/Desktop/SimpleMath-iphonesimulator.xcarchive \
-sdk iphonesimulator \
SKIP_INSTALL=NO
Wait for it complete and you can see the success message.
Then write this very similar command to create another one for iOS devices.
xcodebuild archive \
-scheme SimpleMath \
-archivePath ~/Desktop/SimpleMath-iphoneos.xcarchive \
-sdk iphoneos \
SKIP_INSTALL=NO
After success now you should have the two xcarchives
From there we need to combine both in one xcframework , copy and paste the follow command to your terminal and hit enter
xcodebuild -create-xcframework \
-framework ~/Desktop/SimpleMath-iphonesimulator.xcarchive/Products/Library/Frameworks/SimpleMath.framework \
-framework ~/Desktop/SimpleMath-iphoneos.xcarchive/Products/Library/Frameworks/SimpleMath.framework \
-output ~/Desktop/SimpleMath.
You should get that the xcframwork has been written successfully.
Check your desktop and look for YourFrameworkName.xcframework
Testing the framework
Create a new project from xcode, single view app is okay for testing.
Add your xcframework file you have just created by dragging and dropping it in Framworks, Libraries, and Embedded Content
Now switch over to your view controller and import the framework and start testing it.
Run it on both simulator and real device and it should run and give you the same results.
if you want framework to work with ObjectiveC just add @objc before function or property
Conclusion :
In today’s post, we created a framework, built it, and then successfully added it to a demonstration app. While it seems like we achieved the series’ goal of creating a framework, in reality, we barely scratched the surface and there’s still a lot to learn which we will cover in future post